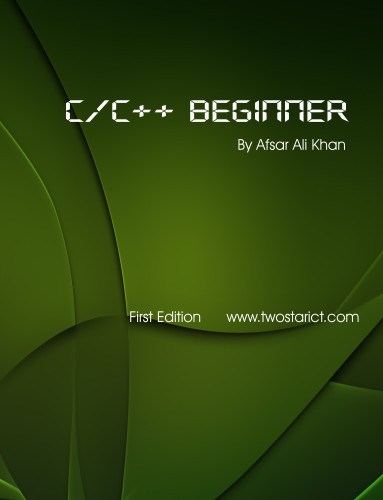
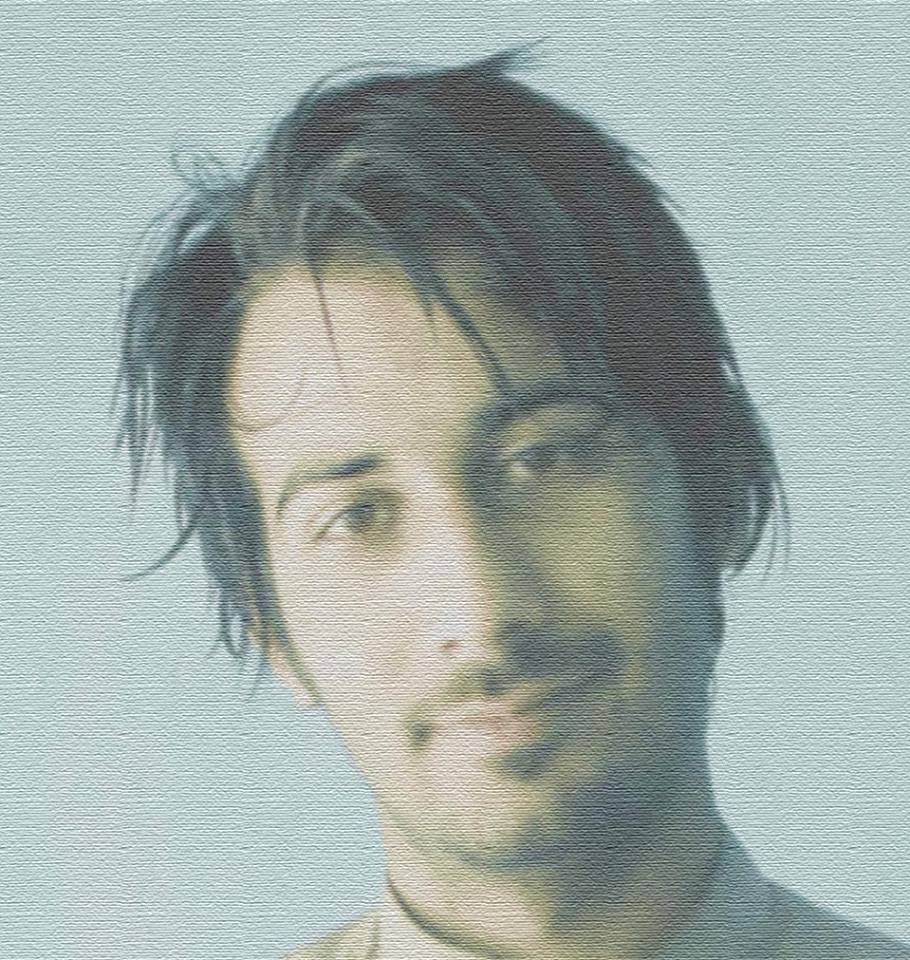
Dedication:
Oh Thanks God! I will like to dedicate my little
effort to my Family, Teachers and Fiends.
Regards:
Afsar Ali Khan
Language
The source of communication between two entities is called Language.
Computer Language.
There are three types of Language used.
High Level Language.
The Language which can easily understandable by humans is called High
Level Language.
Med Level language
The Language which can understandable by both human and machine is
called Med Level Language.
Low Level Language
The Language that can easily understandable by Machine is called Low
Level Language or Machine Language
C Language
C is Med Level Language developed by Dennis Ritche in 1972 at Bells
lab.
C is powerful, flexible, efficient, modular, compiled, and general
purpose.
C is use for any programming tasks.
systems and compilers.
C is rich in operators it has powerful set of operators. There are about
45 operators available in C.
C is structure programming language which leads to clarity, reliability,
error-free and easy to maintain program.
C is close to Hardware.
Computer games is also developed in C its means that C is also use
Graphics and Sound.
C++
C++ was developed by Bjane Stroustrup in 1980 at Bell Lab.
C++ is the superset of C, therefore any legal program in C is a legal C++.
C++ is object oriented programming language.
C++ having many ways of Comments.
C++ support classes that make a program able to hide data.
C++ support Polymorphism. i.e. to create multiple function
C++ was originally called “C with classes”.
Advantages of C
C is used to develop high level Application Programs. ie. Bank
Applications, Scientific Research Application.
C is efficient to for system Application Also because by using C you can
easily define Hardwires.
C is used almost universally.
C Advantages.
C is all purpose programming language. It is suitable for solving
scientific and engineering problems as well as business application. It
can used to develop many low level Applications such as operating
Structure of C/C++ Program.
Each Program in C or C++ contains of the following Basics.
i) Header File
i) Pre-Processor Directives. #include
ii) Source File
iii) Void main Function void main()
iv) Function Body open {
v) Statements;
vi) Function Body Close. }
Some of extra function we will discuss latter on. Let me Take in
Example but before this example if I discuss COMMENT it with pretty
well help you in getting this example.
Comment is Ignore by Compiler. There is a way to write comments in C
but you can use two ways to create comments in C++.
in C we use to write comment in the mid of /* and */
for Example
/* This is Comment */
in C++ you can use the above method too but here is another easy way
to write comment in C++. You just have to write your comment after
double slashes.
and the comment will terminate after you leave that line.
For Example //This is comment.
if you have to write comments in multi line you have to write // before
comment
For Example
// 1st Line of Comment
//Second Line of Comment
//3rd Line of Comment
Now lets take the above structure clearly
#include<soucre_file.h>
main()
{
clrscr();
Statement 1;
Statement 2;
Statement 3
getch();
}
Now Example on the above Method to show “ALI” as output.
//Program to Display name ALI
//This is my First programe
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
cout<<“ALI”;
getch();
}
The Out will be
ALI
Let me discuss the Followed Structure Briefly
Header:
Header is combination of Pre Processor Directives and source file.
Often the First two lines of C programming is Header.
Let me Discuss this two Terms briefly as I can.
i) Pre Processor Directives
ii) Source File
Pre Processor Directives.
It’s not a part of C/C++ programming but it is necessary anyway.
The pre processor Directive tells the compiler to get action and
preprocess the source, by preprocess we mean to process that file
before the program. All the functions that are declared in the source
file pre processed and then the function become workable.
In the above example I had used #include as a pre processor. The
preprocessor directives must be start with #,
Source File:
Source file are that files which are created and kept as a template in
the C/C++ Lab. The Source file contains all the declaration of factions
which we are using in the program.
Source file will be just a path to the header file. Which are located in
the folder INCLUDE of your C/C++ Program.
If you are using the Turbo C++ then the directory to the file will be
C:TCINCLUDE
In the above example the Preprocessor directive #include call the
compiler to include the file conio.h and iostream.h that are located in
the Folder INCLUDE.
In pre processor directives the statement keywords are pre defined.
Just in iostream.h the keyword cout and cin are predefined but if you
use stdio.h than you have to use printf(“aaaaa”) and scanf instead of
cout and cin. Because in stdio.h printf and scanf are predefined.
Main() Function:
When you run a C++ program. The first statement executed will be at
the beginning of a function called main(). It is the part of every C++
program. The program may consist of many functions and classes and
many other program element but on the startup the program will
search for main() function
Function Body:
The function body must be start with { and close with }.
Between this you have to erite the statements. That area is called
function body.
Statements:
In the C or C++ each statement will be written in the function body.
Each Statement will be closed with Semi colon “;”.
You can write a lot of statements.
In the statements we can use different functions also.
Some of them is followed.
Clearscr();
Clearscr(); is used to clears/refresh the screen of out after execution.
If you unfortunately leave clrscr(); you will find the out put same in
number with the number of execution. For example if I run program
once I will see the out put also single but when I run the program again
I will see the out put twice.
Cout<<:
I know pretty well that cout is not so nessary here and I cant discuss
here t briefly, anyhow you must have to know that here I had used as
to display the ALI, instead of cout you can use different member
functions and elements. I will Discuss it briefly after in next topic.
Getch();
Getch() is just use to hold the screen. If you missed the getch(); in
your program the program will run properly but the output screen will
not hold and return you toward the Editor.
Congrats you had learned your first program perfectly and I hope you
will now able to learn more it too easy and interesting. Now you will
learn more function and how o work in C/C++. It will be easy for you.
Working with Veriables
Variables are the most fundamental part of every language. you must
have to know that variables are those that can change their value
during execution.
There are two types of variables using in C/C++
Global variables and Local Variables.
We have to discuss about local variable here.
The construction for the variable in C is that you can Set a value to
local variable everywhere in the function body, but to define variable
we are defining it after the clrscr();
How to Name a Variable?
We must have to write the variable Type and then write the variable
name. The types of variable are limited but you can take any value
according to the rules of C as variable name. The rules for variable
name is that we can use underscore (_) and Alphabets A-Z a-z in the
start of variable name.
The digits etc we can use but not in the start of variable.
E.g. ali _ahmand _123 that all are valued variables.
1ahmad –ali is not a valued variables according to the above rules.
You cant use C++ keywords as variable name. Keyword is a word that is
predefined in the C++ e.g. Class, if, while
This Table show the Variable Types.
Declration Name Type Size Range
Char Character 1Byte -128 to 127
Unsigned char Unsigned character 1Byte 0 to 255
Int Integer 2byte -32768 to 32767
Unsigned int Unsigned integer 2byte 0 to 65535
Signed int Signed integer 2byte -32768 to 32767
Short int Short integer 2byte -32768 to 32767
Signed shot int Signed short integer 2byte -32768 to 32767
Unsigned short int Unsigned short integer 2byte 0 to 65535
Long int Long integer 4byte -2147483648 to 2147483647
Signed long int Signed long integer 4byte -2147483648 to 2147483647
Unsigned long int Unsigned long integer 4byte 0 to 4294967295
Float Floating point 4byte -3.4E+38 to 3.4E+38
Double Double floating point 8byte -1.7E+308 to 1.7E+4932
Long double Long double floating point 10byte -1.7E+4932 to 1.73E+4932
How to assign value to variable?
First we have to write variable name then equal =, then value and then
semi colon;
e.g. a = 5;
and this statement is called Assignment statement.
Now How to Call Variable?
To call variable we we have to write the variable name after cout<< with
out double quote”.
Let me take an example on the above method.
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int a; // Define var
a = 5; // assignment statement
cout<<a; // call var
getch();
}
The out put will be
5
Look deeply to the output I had mentioned a after cout but the result
is 5. It’s mean a was variable and its change its value to 5 as I had
assigned.
Now taking another example of Character variable.
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
char a; // Define var
a = ALI; // assign value
cout<<a; // call var
getch();
}
The output will be
ALI
Oh that’s great. Now going to apply the operator on 2 variables.
#include<iostream.h>
void main();
{
clrscr();
int a, b; // Define var
a = 5; // assign value
b = 10;
cout<<a; // call var
getch();
}
The out put will be
15
Okay now going to clear that cout<< is used to display output
You know that variable hold a value until the program is closed?.
Here is another keyword cinn>> by which we can enter a vale from
keyboard in out put.
In the following example we will show to the user to enter value for a
and then enter vale for b and the program will add both values.
When user enter the value for a the variable a hold the value of user
until the program restart, and in the same way for b as will.
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int a, b, c; // Define var
cout<<“Enter 1st value”; // Just show to the user
cinn>>a; // Assign value for the user keyboard to var a
cout<<“Enter 2nd value”;
cin>>b;
c = a+b; //Define the C var
cout<<“result”<<c; //show var c as output
getch();
}
The out put will be
Enter 1st value15 Enter 2nd vale10 result 25
Now going to use another interesting keyword endl; which will end the
line in output.
Same above example.
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int a, b, c; // Define var
cout<<“Enter 1st value”<<endl; // Just show to the user
cinn>>a<<endl; // Assign value for the user keyboard to
// var a
cout<<“Enter 2nd value”<<endl;
cin>>b<<endl;
c = a+b<<endl; //Define the C var
cout<<“result”<<c; //show var c as output
getch();
}
The output will be
Enter 1st value
15
Enter 2nd vale
10
result 25
That’s all we done are statements. Because it close with semi colon.
Hope you had got the structure of above programs and understand
working wit variable.
Arithmetic Operators:
There are 5 arithmetic operators using in C/C++
OPERATOR PURPOSE
+ Addition
– Subtraction
* Multiplication
/ Division
% Reminder Operator
Explanation Examples
2+2 = 4
4-2 = 2
10/5 = 2
2*5 = 10
2%2 = 0
The Priority of Operations:
i) All parentheses are evaluated first.
ii) Multiplication, Division and Modulus are performed next.
iii) Addition and subtraction will be performed next
Few examples below.
i) 4+3*3-6 = 7
ii) (4+3)*3-6 = 15
iii) 5+5%3 = 7
How to assign string Data?
A string is simply the list of one or more characters. In C++, strings
are stored in an array of characteristics.
Bracket [] is used to declare string characters.
For example
Char Name[10] = “Ali khan”
Keep remember that Ali Khan is the combination of 8 characters so it
is valued but if you want to assign ALI KHAN AFRIDI so it will be not a
valued value. Because it is the combination of 15 words.
In the above example I had assigned the string data to variable Name
of about 10 characters. So let me explain this with a table. Take a
table of 10 cells and enter 1 word of the name to each cell.
A L I K H A N
Now how to write program for string data?
#include<conio.h>
#include<string.h>
void main();
{
clrscr();
char Name[10];
strcpy(Name, “ALI KHAN”);
cout<<“My Name is “<<Name;
getch();
}
The out put will be
ALI KHAN
Decision Making Statements:
Same here we are taking decision on many ways. You had ever seen that
a body got less than 33% marks so it is considered as fail. When he
told you their marks so you start thinking to take a decision that as he
passed or fail.
Same in the way you can take decision in C or C++.
Here are few Types of Decision making statements.
The IF Statement:
In the if statement you are able to take decision on one way that as
the person passed?
Syntax for the If Statement
If(condition)
Statement;
And for multiple statements
If(condition)
{
Statement 1;
Statement 2;
.
.
.
Statement n;
}
Now take an Example in the program
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int a;
a=15;
if(a<=30)
cout<<“Fail”;
getch();
}
The output will be
Fail
If you change the value form 15 to 33 then the program will return to
the C++ editor.
Now example to Show multiple statements.
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int a;
a=15;
if(a<=30)
{
Cout<<’x07’; //Beep
cout<<“Fail”<<endl;
cout<<“Poor Result”;
getch();
}
The Out put will be with a beep
Fail
Poor Result
Now we are going to get valu from the user and ask compiler to
decision over it.
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int marks;
cout<<“Enter your Marks”<<endl;//Display user to Enter
//Marks
Cin>>marks; // Assign the user value to var marks
if(marks<=33)
{
Cout<<’x07’; //Beep
cout<<“Fail”<<endl;
cout<<“Poor Result”;
getch();
}
Now You have to enter your marks and this program will decide that
have you a poor result.
IF-Else Statements:
In the if-else statements there are two routs in the decision that if
the condition true? When the condition is true then the program will
give the statement after condition other wise the program will give you
the statement after else.
Syntax
If(condition){
Statement;
}Else{
Statement;
}
And for multiple statements
If(condition)
{
Statement 1;
Statement 2;
Statement 3;
}
Else
{
Statement 1;
Statement 2;
Statement 3;
}
Now let me take example in C/C++
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int marks;
cout<<“Enter your Marks”<<endl;//Display user to Enter
//Marks
Cin>>marks; // Assign the user value to var marks
if(marks<=33)
{
Cout<<’x07’; //Beep
cout<<“Fail”<<endl;
cout<<“Poor Result”;
}else{
Cout<<“Passed”<<endl;
Cout<<“Congrats”;
getch();
}
Now when you enter the value less then 33 the out put will with beep
Enter your Marks
15
Fail
Poor Result
And if you enter the value greater than 33 then the output will be
passed
Congrats
The Nested if-else statements:
If-else statement within another if-else statement is called Nested if
Statement
The structure of Nested if statement will be
If(condition){
If(condition){
Statement1;
}Else{
Statement2;
}Else{
Statement3;
}
}
Keep in mind that for the first if statement the last else will be
performed and for the 2nd if the 2nd last else will be performed. And so
on.
For example I am going to write a program that if the marks is greater
than 33 then Show the result with Good or Satisfactory otherwise
Show Fail.
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int marks;
cout<<“Enter your Marks”<<endl;//Display user to Enter
//Marks
Cin>>marks; // Assign the user value to var marks
if(marks>33){
if(marks>50){
cout<<“Good”;
}else{
cout<<“Satisfactory”;
}else{
cout<<“Fail”;
cout<<“Marks = “<<marks;
}
getch();
}
Now when you Enter 53 the result will be
Enter your Marks
53
Good
Marks = 53
And when you enter 45 then the output will be
Enter your Marks
45
Satisfactory
Marks = 45
And when you enter 15 then the output will be
Enter your Marks
15
Fail
Marks = 15
The Switch Statement:
It is also called multiple choice statements. Here must be 3 things.
Case: That will check your choice.
Break: which your choice wills match the case then the program will
break. And don’t check the below program.
Default: when your choices do not match with case then it will go the
default.
Syntax:
Switch(expression)
{
Case valueOne: statement;
Break;
Case valueTwo: statement;
Break;
:
:
:
Case ValueN: statement(s);
Break;
Default: statement;
}
Now going to write a program.
In this program you will asked to enter your choice 1 for Prgramer
Name
2 for Programmer Cell Number
And 3 for Programmer Detail.
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int choice;
cout<<“******************”;
cout<<“Enter your Choice”<<endl;
cout<<“1 ******** Name”<<endl;
cout<<“2 ******** Cell No”<<endl;
cout<<“3 ******** Detail”<<endl;
cout<<“******************”;
cin>>choice;
switch(choice)
{
case 1: cout<<“Programmer name is Afsar Ali Khan”;
break;
case 2: cout<<“The Mobile Number of Programmer is
00000000000″;
break;
case 3: cout<<“MCS and CTO at Two Star”;
break;
default: cout<<“Your Entered choice was not correct;
break;
getch();
}
Let me explain this method in another way. Want to create a calculator
in this way.
The operator +, -, *, / will used to perform action on two values. If a
user input a wrong operator the program will show to user that you’re
entered operator didn’t found,
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
char op;
int x,y;
cout<<“******************”;
cout<<“Enter value for X “<<endl;
cin>>x<<endl;
cout<<“******************”<<endl;
cout<<“Enter value for Y”<<endl;
cin>>y<<endl;
cout<<“******************”<<endl;
cout<<“Enter Operator + – / * “;
cin>>op<<endl;
cout<<“******************”;
switch(op)
{
case ‘+’: cout<<x+y;
break;
case ‘-‘: cout<<x-y;
break;
case ‘*’: cout<<x*y;
break;
case ‘/’: cout<<x/y;
break;
default: cout<<“Your Entered Operator not found;
break;
getch();
}
Loops:
The repetition of statements is called Looping. Or it is a repeatitive
statement which executes a statement or block of statements again
and again. There are three types of loop in C++.
i) While Loop.
ii) Do while Loop
iii) For Loop.
For Loop:
The for loop is control by 3 parts:
a) Initialization
b) Continuation condition
c) Update
Syntax
For(initialization; continuous; update)
{
Statement1;
Statement2;
}
For Example:
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int a;
for(a=0; a<=10; a++)
{
cout<<a;
}
getch();
}
The Out put will be
12345678910
Now when we have to show each digit in other row.
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int a;
for(a=0; a<=10; a++)
{
cout<<a<<endl;
}
getch();
}
The out put will be
1
2
3
4
5
6
7
8
9
10
Now I am just going to show Pakistan 10 time using Loop.
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int a;
for(a=0; a<=10; a++)
{
cout<<“PAKISTAN”<<endl;
}
getch();
}
The out put will be
PAKISTAN
PAKISTAN
PAKISTAN
PAKISTAN
PAKISTAN
PAKISTAN
PAKISTAN
PAKISTAN
PAKISTAN
PAKISTAN
While Loop:
While loop is used when it is not known in advance that how many time
the loop will repeat.
Syntax:
While(condition){
Statemnt1;
Stament2;
}
For Example:
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int n=1, sum=0;
while(n<=100)
{
sum+=n;
n++;
cout<<“Sum of 1st 100 number = “<<sum;
}
getch();
}
The Do-While Loop:
It is different from for and while loop.
Body of the loop is executed first and then the condition is checked
Body of the loop must be executed if the condition become false.
Syntax:
Do{
Statment1;
Statment2;
}
While(condition);
For Example:
#include<conio.h>
#include<iostream.h>
void main();
{
clrscr();
int n, f=1;
cout<<“Enter a number”<<endl;
cin>>n<<endl;
cout<<“Factorial is “;
do{
f*=n;
n–;
}
Whie(n>1)
Cout<<f<<endl;
getch();
}
okay man!!!!
I hope you had learned C/C++ beginner level.
that’s all people learned in initial stages, I wish you happy and successful journey in the field of Computer Programming….
Let me know some thing from you perople
Study make a man _________________ ?
Write answer after studying this book
Published: May 10, 2015
Latest Revision: May 10, 2015
Ourboox Unique Identifier: OB-33991
Copyright © 2015
You are really great personality sir I like you always like and like you forever
Good job sir